
Something needs to hold on to the intermediate state, otherwise the app won’t really do much of anything.
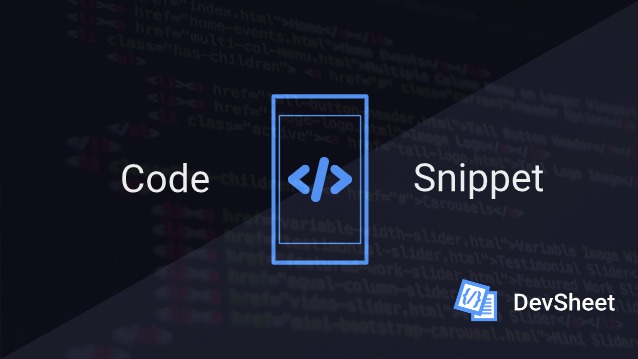
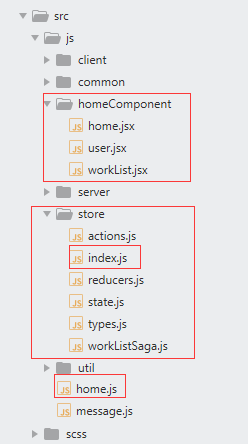
#REDUX REDUCER DRIVER#
Now, the downside with a function like this is that it needs a driver of sorts.
#REDUX REDUCER CODE#
It is easy to figure out what it does by reading its code (and easy to debug!) because it’s all self-contained. It doesn’t matter what else has changed in your app – this function will always act the same way.
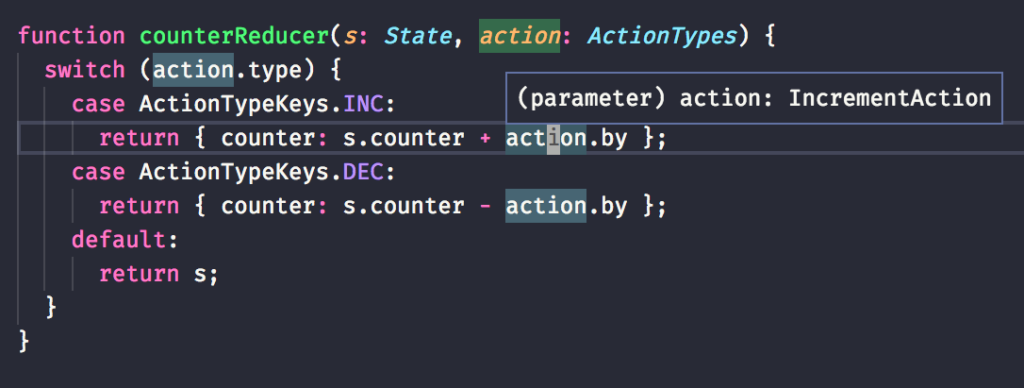
If you call it with the same arguments, you get the same outputs, every single time. I’ll tell you: This function is nice because it is predictable. What good is a function that doesn’t remember anything, and doesn’t change anything? I can tell you don’t think this is very useful. Notice that the reducer has an initial state (when given undefined, it returns an empty string anyway), and that it has a default case that handles any actions it doesn’t understand (it returns the existing state, unchanged, when it sees such an action). reduce ( function ( accumulatedResult, arrayItem ) )) // => 'existing state' All of the commands except eject will still work, but they will point to the copied scripts so you can.
#REDUX REDUCER FULL#
Instead, it will copy all the configuration files and the transitive dependencies (webpack, Babel, ESLint, etc) right into your project so you have full control over them. Var letters = // `reduce` takes 2 arguments: // - a function to do the reducing (you might say, a "reducer") // - an initial value for accumulatedResult var word = letters. This command will remove the single build dependency from your project. This will make more sense with an example: Your function gets called with 2 arguments: the last iteration’s result, and the current array element. It takes a function as an argument, and it calls your provided function once for each element of the array, similar to how Array.map works (or a for loop, for that matter). ( Technically I should be writing it as, because it is a function on array instances, not on the capital-A Array constructor.) JavaScript’s Array has a built-in function called reduce. If you aren’t yet familar with Array.reduce, here’s what’s up: And if you know Array.reduce, you know that it takes a function (one might call it a “reducer” function) that has the signature (accumulatedValue, nextItem) => nextAccumulatedValue.
#REDUX REDUCER UPDATE#
It uses Immer internally to drastically simplify immutable update logic by writing 'mutative' code in your reducers, and supports directly mapping specific action types to case reducer functions that will update the state when that action is dispatched. It might not seem too foreign if you’re familiar with functional programming and JavaScript’s Array.reduce function. A utility that simplifies creating Redux reducer functions. It might seem a bit scary and foreign, but after this short article I think you’ll come to agree that it is, as the saying goes, “just a function.”įirst off, where does the name “reducer” come from? Redux didn’t actually make it up (I was kidding about that). The action specifies what occurred, and the reducer’s role is to return the updated state as a result of that action. One of those things is what a reducer is and what it does. A reducer is a pure function in Redux that accepts an action and the application’s previous state and returns the new state. In order to work with Redux, you need to know a few things. It applies middlewares to store.Reducer, n. Redux provides with API called applyMiddleware which allows us to use custom middleware as well as Redux middlewares like redux-thunk and redux-promise.
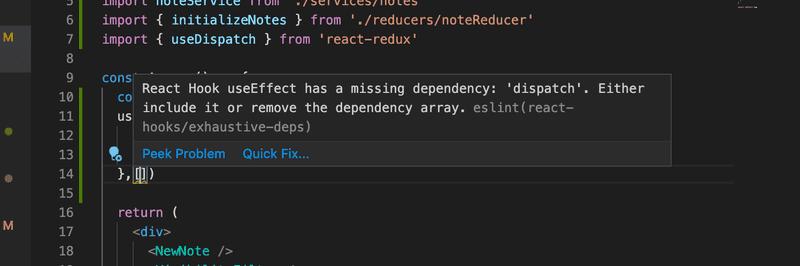
You can imagine middlewares somewhere between action dispatched and reducer.Ĭommonly, middlewares are used to deal with asynchronous actions in your app. Which allows you to get state anywhere in your code like so: const localState1 getState (reducerA.state1) const localState2 getState (reducerB.state2) But think first if it would be better to pass the external state as a payload in the action. Multiple middlewares can be combined together to add new functionality, and each middleware requires no knowledge of what came before and after. Section 4: Reusing common reducers between different places in state Section 5: Best practices for connecting React components to Redux state. You can try to use: redux-named-reducers. Customized middleware functions can be created by writing high order functions (a function that returns another function), which wraps around some logic. In this case, Redux middleware function provides a medium to interact with dispatched action before they reach the reducer. Reducer has nothing to do with who performs it, how much time it is taking or logging the state of the app before and after the action is dispatched. As discussed earlier, reducers are the place where all the execution logic is written. Redux itself is synchronous, so how the async operations like network request work with Redux? Here middlewares come handy.
